Docker Concepts & Collection of Handy Commands
- Published on
- • 6 mins read
What is Docker ?
Docker is an open platform for developing, shipping, and running applications, which enables you to separate your applications from your infrastructure so you can deliver software quickly and solve the problem of "But, It works on my machine...".
Docker container :-
- A way to package application with all necessary dependencies and configurations.
- Isolated place where an application runs without affecting the rest of the system and without the system impacting the application.
Docker Image :-
- Read-only templates containing instructions for creating a container. (i.e. Blueprint or snapshot of what will be in container when it runs)
- Artifact that can be moved around and relies on the host operating system (OS) kernel.
- You can manually build images using a Dockerfile (covered later), a text document containing all the commands to create a Docker image.
Docker Image Commands :-
# Pulls the docker image form repository (e.g. Docker Hub)
docker pull <IMAGE_NAME>
# pulls latest version of image (this is default behavious of docker pull command)
docker pull <IMAGE_NAME>:latest
# pulls specified version of the image (i.e. In this case, 9.6.4)
docker pull <IMAGE_NAME>:9.6.4
# To check existing images on host/local machine
docker images
# Running the container of specified image in attached mode
docker run <IMAGE_NAME>
# Running the container in detached mode
docker run -d <IMAGE_NAME>
# Removes pulled docker image from host machine
docker rmi <IMAGE_ID>
Docker Container Commands :-
# To check all the running instances of docker containers on host machine
docker ps
# Listing all the containers on host machine (even the non-running ones)
docker ps -a
# Stops the running container
docker stop <CONTAINER_ID>
# Starts the stopped container
docker start <CONTAINER_ID>
# Removes docker container from host machine
docker rm <CONTAINER_ID>
Container Port vs Host Port :
- Within container (i.e. the isolated environment of your packaged application) application/services runs on some port which is called the Container Port
- By default when we run our container, same port number of container port is used on the host machine (Host Port) as well.
- Now, Host machine can have multiple containers running on it, And we can get conflict when different containers expect same port on the host machine.
- To solve this issue, use concept of port forwarding -
docker run -p <HOST_PORT>:<CONTAINER_PORT> <IMAGE_NAME>
Commands continued :-
# Checking the logs of the container to debug the application
docker logs <CONTAINER_ID> # OR <CONTAINER_NAME>
# Assigning name to the container
docker run -d -p <HOST_PORT>:<CONTAINER_PORT> --name <CONTAINER_NAME> <IMAGE_NAME>
Docker Network :
- Docker creates its isolated docker network where multiple containers can run in.
- Containers within the same docker network can talk to each other by just container name. i.e. their complete addresses (e.g. localhost, port, etc.) not needed.
- One container can be part of multiple docker networks
# Lists the docker networks created on host/local machine
docker network ls
# Creates new docker network
docker network create <NETWORK_NAME>
Running container within docker network :-
docker run -d \
-p <HOST_PORT>:<CONTAINER_PORT \
-e <ENV_VARIABLE>=<ENV_VALUE>
--name <CONTAINER_NAME> \
--net <NETWORK_NAME> \
<IMAGE_NAME>
Note -
-e
- To define environment variables for application running in container--net
- Defines docker network name within which we want to run our container--name
- Assigns container name\
(back slash) - To divide the long command over multiple lines
Dockerfile :-
- Blueprint for creating your own docker images.
- It's a text document (named
Dockerfile
only) that contains all the commands a user could call on the command line to assemble an image. - Example -
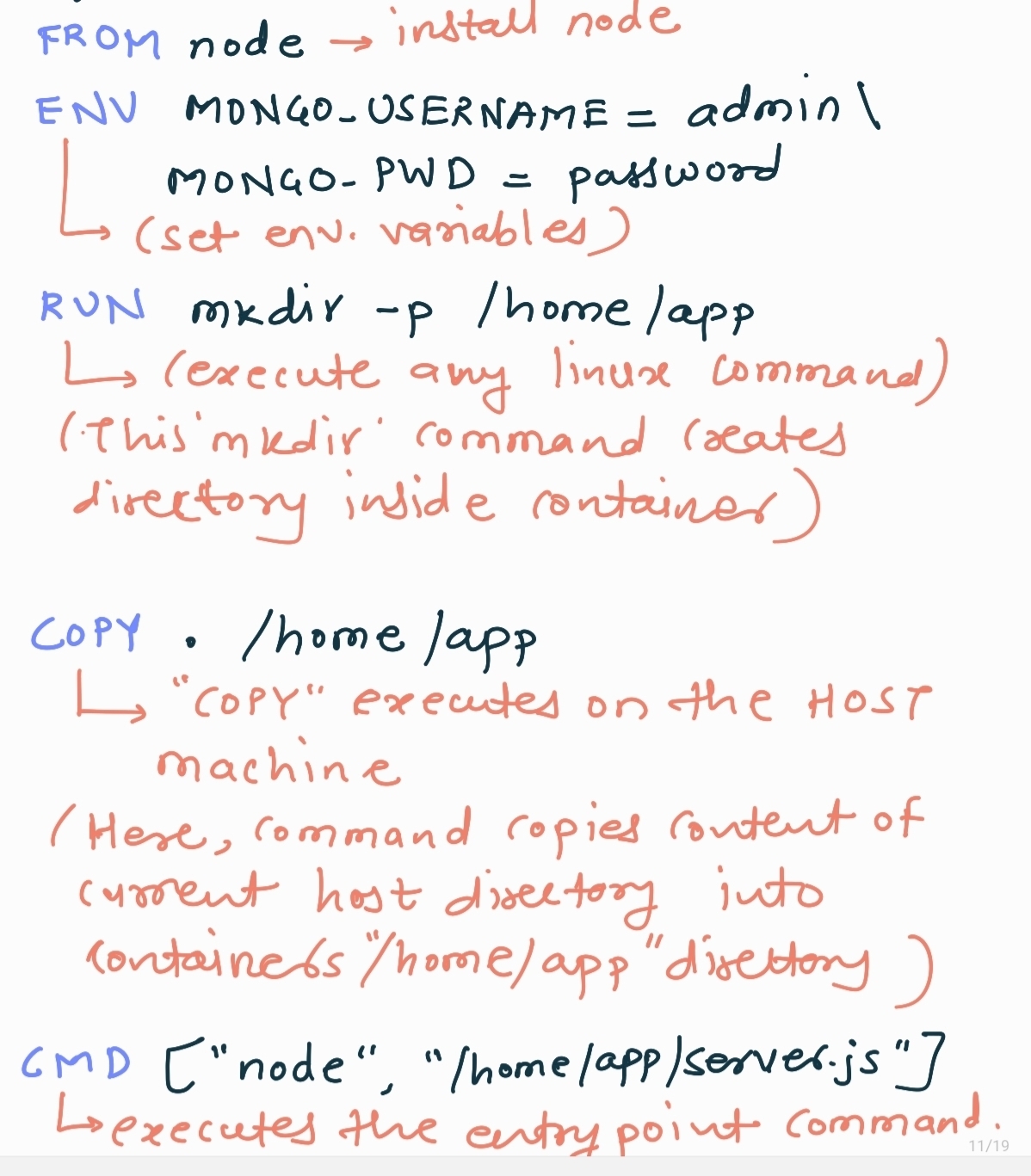
Docker Volumes :-
- Preferred mechanism for persisting data generated by and used by Docker containers.
- Generally when container stops/removed/restarted, the data is gone. So if we want to keep data between run, docker volumes are used.
- Folder in physica host filesystem is mounted into the virtual filesystem of docker container
- Data gets automatically replicated so even when container stops/restarts it gets data from host filesystem.
3 Types of Volumes :
- Host Volumes - You decide where on the host filesystem the reference is made.
Example -
docker run -v /home/mount/data:/var/lib/mysql/data # before colon, host directory and after, container directory
- Anonymous Volumes - You don't specify directory on host filesystem. Docker takes care of automatically creating directory under
/var/lib/docker/volumes/
.
# Here only specifying container directory
docker run -v /var/lib/mysql/data <IMAGE_NAME>
- Named Volumes - Improvement over anonymous volumes. Here we reference volume by name, Therefore we don't need to know exact host directory path.
docker run -v <VOL_NAME>:/var/lib/mysql/data <IMAGE_NAME>
Docker Compose :
- Tool to help define and share multi-container applications
- We create YAML file (called
docker-compose.yml
) to define the services & with single command can spin everything up or tear it down - Docker compose takes care of creating a common network by default.
# Starts all the containers defined in docker-compose.yml
docker compose up
# Removes all containers as well as network created by docker compose
docker compose down
Note - In case you are not using default name docker-compose.yml, then you have to specify the docker compose file name as well in the command using -f
flag. e.g. docker compose -f <FILE_NAME.yml> up
Example -
docker-compose.yml
version: '3'
services:
web:
# Path to dockerfile.
# '.' represents the current directory.
build: .
# Mapping of container port to host
ports:
- "5000:5000"
# Mount volume
volumes:
- "/usercode/:/code"
# Link database container to app container for reachability.
links:
- "database:backenddb"
database:
# image to fetch from docker hub
image: mysql/mysql-server:5.7
# define container name
container_name: app_mysql
# Environment variables for startup script
# container will use these variables to start the container with these defined variables.
environment:
- "MYSQL_ROOT_PASSWORD=rootpass"
- "MYSQL_USER=root"
- "MYSQL_PASSWORD=pass"
- "MYSQL_DATABASE=backend"